Verify - Send 2FA SMS
Send SMS with verification codes.
Available HTTP Methods: POST

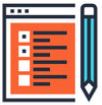
Parameters
Parameter | Type | Description | Required |
---|---|---|---|
message | string | JSON string with the variables to send a SMS. | Yes |
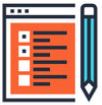
Message JSON
{ "messagetext" : "This your code", "senderid" : "Sendmode", "recipient" : "0870000000" }
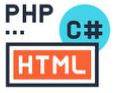
Code Examples
C#
using (WebClient client = new WebClient()) { client.Headers.Add("Authorization", "YOUR_ACCESS_KEY"); var postJson = new JavaScriptSerializer().Serialize(new { messagetext = "This is your code.", senderid = "Sendmode", recipient = "0870000000" } ); byte[] response = client.UploadValues("https://rest.sendmode.com/v2/verify", new NameValueCollection() { { "message", postJson } }); string result = System.Text.Encoding.UTF8.GetString(response); }
php
$arr = array('messagetext' => 'This is a test', 'senderid' => 'SendMode', 'recipient' => '0870000000'); $url = 'https://rest.sendmode.com/v2/verify'; $data = array('message' => json_encode($arr)); // use key 'http' even if you send the request to https://... $options = array( 'http' => array( 'header' => "Content-type: application/x-www-form-urlencoded\r\n" . "Authorization: YOUR_ACCESS_KEY\r\n", 'method' => 'POST', 'content' => http_build_query($data) ) ); $context = stream_context_create($options); $result = file_get_contents($url, false, $context);
Java
HttpClient httpclient = HttpClients.createDefault(); HttpPost httppost = new HttpPost("https://rest.sendmode.com/v2/verify"); httppost.setHeader(HttpHeaders.AUTHORIZATION, YOUR_ACCESS_KEY); String json = "{\"messagetext\" : \"Hello World\", \"recipient\" : \"0870000000\"}"; List<NameValuePair> params = new ArrayList<NameValuePair>(1); params.add(new BasicNameValuePair("message", json)); httppost.setEntity(new UrlEncodedFormEntity(params, "UTF-8")); HttpResponse response = httpclient.execute(httppost); HttpEntity entity = response.getEntity();

Response
{ "status":"OK", "statusCode":0, "acceptedDateTime":"2017-08-29T09:23:47.4120695+01:00", "message": { "senderid":"Sendmode", "messagetext":"This is your code", "recipient":"0852455041", "tokenLength":0, "timeout":0 } }
Verify - Verify Token
Verify the token sent in your 2FA SMS.
Available HTTP Methods: POST

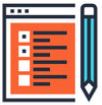
Parameters
Parameter | Type | Description | Required |
---|---|---|---|
recipient | string | Mobile number of recipient of 2FA SMS. | Yes |
code | string | The 2FA token received in the 2FA SMS. | Yes |
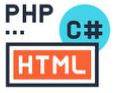
Code Examples
C#
using (WebClient client = new WebClient()) { client.Headers.Add("Authorization", "YOUR_ACCESS_KEY"); byte[] response = client.UploadValues("http://rest.sendmode.com/v2/verify", new NameValueCollection() { { "code", "YOUR_VERIFY_CODE" }, { "recipient", "0870000000"} }); string result = System.Text.Encoding.UTF8.GetString(response); }
php
$url = 'https://rest.sendmode.com/v2/verify'; $data = array('code' => '2FA_CODE', 'recipient' => '0870000000'); // use key 'http' even if you send the request to https://... $options = array( 'http' => array( 'header' => "Content-type: application/x-www-form-urlencoded\r\n" . "Authorization: YOUR_ACCESS_KEY\r\n", 'method' => 'POST', 'content' => http_build_query($data) ) ); $context = stream_context_create($options); $result = file_get_contents($url, false, $context);
Java
HttpClient httpclient = HttpClients.createDefault(); HttpPost httppost = new HttpPost("https://rest.sendmode.com/v2/verify"); httppost.setHeader(HttpHeaders.AUTHORIZATION, YOUR_ACCESS_KEY); List<NameValuePair> params = new ArrayList<NameValuePair>(2); params.add(new BasicNameValuePair("code", "2FA_CODE")); params.add(new BasicNameValuePair("recipient", "0870000000")); httppost.setEntity(new UrlEncodedFormEntity(params, "UTF-8")); HttpResponse response = httpclient.execute(httppost); HttpEntity entity = response.getEntity();

Response
{ "status":"OK", "statusCode":0, "message":{ "recipient":"353852455041", "code":"78b6", "expireDatetime":"29/08/2017 13:42:52", "status":"VERIFIED", "verifiedDate":"29/08/2017 11:43:31" } }